6.3. Inversion with a configuration file¶
In this notebook we show how to use a configuration file to run Hazel in different situations for inverting Stokes profiles.
6.3.1. On-disk inversions¶
Let’s first a set of observations obtained from the GREGOR telescope as example. The observations consisted of a scan of an active region in which filaments are seen when observed in the core of the He I 10830 A line.
[1]:
%matplotlib inline
import numpy as np
import matplotlib.pyplot as pl
import hazel
import h5py
import scipy.io as io
print(hazel.__version__)
label = ['I', 'Q', 'U', 'V']
2018.9.22
First read the observations and do some plots. The wavelength axis in the save file is given in displacement with respect to some reference wavelength, in this case 10830.0911 A.
[2]:
tmp = io.readsav('/scratch/Dropbox/test/test_hazel2/orozco/gregor_spot.sav')
print(tmp.keys())
f, ax = pl.subplots(nrows=1, ncols=2, figsize=(10,6))
ax[0].imshow(tmp['heperf'][:,0,:,0])
ax[1].imshow(tmp['heperf'][:,0,:,181])
f, ax = pl.subplots(nrows=2, ncols=2, figsize=(10,10))
stokes = np.zeros((4,210))
stokes[0,:] = tmp['heperf'][160,0,130,0:-40] / np.max(tmp['heperf'][160,0,130,:])
stokes[1,:] = tmp['heperf'][160,1,130,0:-40] / np.max(tmp['heperf'][160,0,130,:])
stokes[2,:] = tmp['heperf'][160,2,130,0:-40] / np.max(tmp['heperf'][160,0,130,:])
stokes[3,:] = tmp['heperf'][160,3,130,0:-40] / np.max(tmp['heperf'][160,0,130,:])
ax[0,0].plot(tmp['lambda'][0:-40] + 10830.0911, stokes[0,:])
ax[0,1].plot(tmp['lambda'][0:-40] + 10830.0911, stokes[1,:])
ax[1,0].plot(tmp['lambda'][0:-40] + 10830.0911, stokes[2,:])
ax[1,1].plot(tmp['lambda'][0:-40] + 10830.0911, stokes[3,:])
wvl = tmp['lambda'][0:-40]
stokes = stokes[:,:]
n_lambda = len(wvl)
print(n_lambda)
dict_keys(['heperf', 'lambda'])
210
/scratch/miniconda3/envs/py36/lib/python3.6/site-packages/matplotlib/figure.py:2362: UserWarning: This figure includes Axes that are not compatible with tight_layout, so results might be incorrect.
warnings.warn("This figure includes Axes that are not compatible "
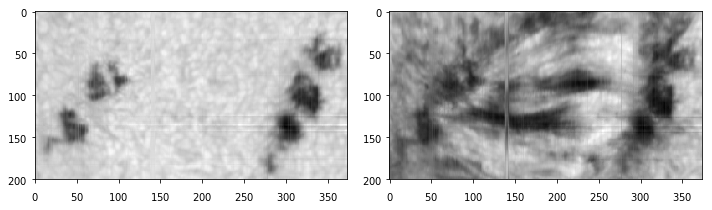
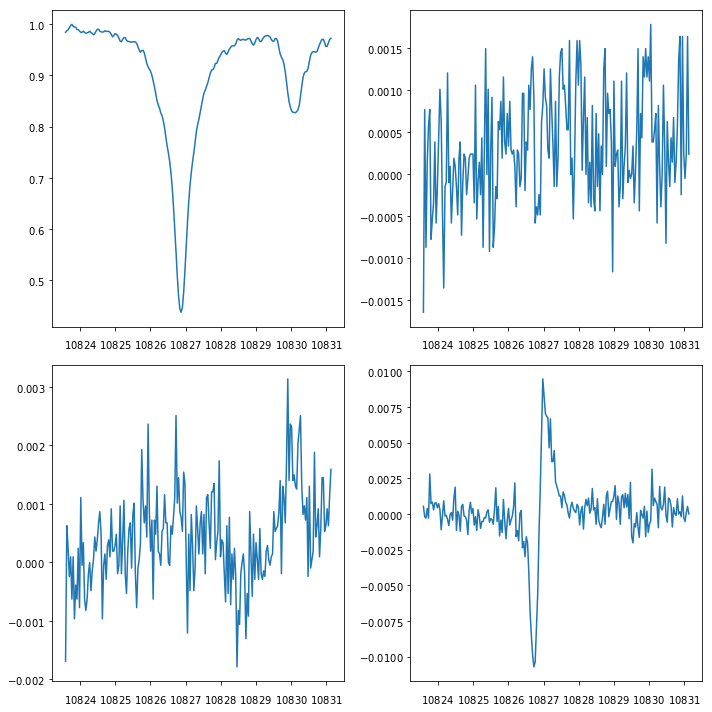
Let’s compute a very rough estimation of the noise standard deviation:
[3]:
noise = np.std(stokes[1,0:20])
Now we save 1D files for the inversion. You can use the tools provided by the code, which makes things really easy:
[4]:
tmp = hazel.tools.File_observation(mode='single')
tmp.set_size(n_lambda=n_lambda, n_pixel=1)
print(tmp.obs['stokes'].shape)
tmp.obs['wavelength'] = wvl+10830.0911 # The wavelength in the file is given wrt the blue component but this is wrong
tmp.obs['stokes'][0,:,:] = stokes.T
tmp.obs['sigma'] = noise*np.ones((n_lambda,4))
tmp.obs['los'][0,:] = np.array([0.0,0.0,90.0])
tmp.obs['boundary'][:] = 0.0
tmp.obs['boundary'][0,:,0] = 1.0
tmp.obs['weights'][:] = 1.0
tmp.save('10830_spot')
(1, 210, 4)
Saving wavelength file : 10830_spot.wavelength
Saving weights file : 10830_spot.weights
Saving 1D Stokes file : 10830_spot.1d
You can also do it by hand, which is not so easy to understand:
[5]:
np.savetxt('10830_spot.wavelength', wvl+10830.0911, header='lambda')
f = open('10830_spot.weights', 'w')
f.write('# WeightI WeightQ WeightU WeightV\n')
for i in range(n_lambda):
f.write('1.0 1.0 1.0 1.0\n')
f.close()
f = open('10830_spot.1d', 'wb')
f.write(b'# LOS theta_LOS, phi_LOS, gamma_LOS\n')
f.write(b'0.0 0.0 90.0\n')
f.write(b'\n')
f.write(b'# Boundary condition I/Ic(mu=1), Q/Ic(mu=1), U/Ic(mu=1), V/Ic(mu=1)\n')
f.write(b'1.0 0.0 0.0 0.0\n')
f.write(b'\n')
f.write(b'# SI SQ SU SV sigmaI sigmaQ sigmaU sigmaV\n')
tmp = np.vstack([stokes, noise*np.ones((4,n_lambda))])
np.savetxt(f, tmp.T)
f.close()
[6]:
%cat 10830_spot.1d
# LOS theta_LOS, phi_LOS, gamma_LOS
0.0 0.0 90.0
# Boundary condition I/Ic(mu=1), Q/Ic(mu=1), U/Ic(mu=1), V/Ic(mu=1)
1.0 0.0 0.0 0.0
# SI SQ SU SV sigmaI sigmaQ sigmaU sigmaV
9.844778180122375488e-01 -1.641324721276760101e-03 -1.689598895609378815e-03 5.310168489813804626e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.873363971710205078e-01 7.723881280981004238e-04 6.275653140619397163e-04 -1.930970320245251060e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.892690181732177734e-01 -8.689365931786596775e-04 2.413712936686351895e-04 -2.896455407608300447e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.933860301971435547e-01 1.448227703804150224e-04 -2.413712936686351895e-04 3.861940640490502119e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.975462555885314941e-01 5.792910815216600895e-04 9.654849418438971043e-05 -2.896455407608300447e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
1.000000000000000000e+00 7.723881280981004238e-04 -6.275653140619397163e-04 2.799906767904758453e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.961368441581726074e-01 -7.723881280981004238e-04 9.654850873630493879e-05 7.241138373501598835e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.948430061340332031e-01 -5.310168489813804626e-04 -9.654851746745407581e-04 8.206623606383800507e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.940773248672485352e-01 -3.379197733011096716e-04 -3.861940640490502119e-04 2.896455407608300447e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.898141622543334961e-01 3.861940640490502119e-04 -6.275653140619397163e-04 7.723881280981004238e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.895681738853454590e-01 -5.792910815216600895e-04 2.413712936686351895e-04 7.723880698904395103e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.867938160896301270e-01 -1.448227703804150224e-04 -7.723881280981004238e-04 4.344683256931602955e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.842048287391662598e-01 4.344682965893298388e-04 1.110307872295379639e-03 7.241138373501598835e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.844613075256347656e-01 1.013759407214820385e-03 -4.827425800613127649e-05 2.896455407608300447e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.865425229072570801e-01 6.275653140619397163e-04 3.379198315087705851e-04 -1.110307872295379639e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.840631484985351562e-01 -4.827425873372703791e-04 -6.275653140619397163e-04 -9.654851601226255298e-05 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.825372099876403809e-01 -1.351679093204438686e-03 -8.206623606383800507e-04 9.172108839266002178e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.832659959793090820e-01 -1.448227703804150224e-04 -6.275653140619397163e-04 -1.448227703804150224e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.843139052391052246e-01 -9.654852328822016716e-05 -1.930970320245251060e-04 -9.654849418438971043e-05 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.864993095397949219e-01 1.206856337375938892e-03 0.000000000000000000e+00 -3.861940640490502119e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.839375019073486328e-01 -9.654851601226255298e-05 -4.827425873372703791e-04 -8.206623606383800507e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.821277856826782227e-01 9.654851601226255298e-05 -1.448227703804150224e-04 -4.827424709219485521e-05 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.795750975608825684e-01 -5.792910815216600895e-04 4.827425436815246940e-05 9.654851601226255298e-05 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.815246462821960449e-01 -1.930970320245251060e-04 4.344682965893298388e-04 -4.344682965893298388e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.866189360618591309e-01 1.930970320245251060e-04 1.930970320245251060e-04 1.110307872295379639e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.899973273277282715e-01 9.654850873630493879e-05 3.861940349452197552e-04 1.882695942185819149e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.903193116188049316e-01 -1.448227703804150224e-04 6.758395466022193432e-04 -1.158582163043320179e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.866821169853210449e-01 -4.827425873372703791e-04 8.689365931786596775e-04 1.930970320245251060e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.851867556571960449e-01 1.930970320245251060e-04 4.827425873372703791e-04 -9.654851601226255298e-05 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.847785830497741699e-01 3.861940349452197552e-04 -9.654851746745407581e-04 -1.206856337375938892e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.852656126022338867e-01 -7.241138373501598835e-04 -4.827424709219485521e-05 5.310168489813804626e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.872715473175048828e-01 -9.654852328822016716e-05 1.448227703804150224e-04 6.758395466022193432e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.860650300979614258e-01 2.413712936686351895e-04 -2.896455407608300447e-04 -1.448227558284997940e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.862878918647766113e-01 1.930970320245251060e-04 2.896455407608300447e-04 -1.930970465764403343e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.861755967140197754e-01 -2.413712936686351895e-04 3.861940349452197552e-04 -4.344682965893298388e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.841112494468688965e-01 -4.827426164411008358e-05 9.654851601226255298e-05 -1.448227674700319767e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.802458882331848145e-01 1.930970320245251060e-04 9.172108839266002178e-04 2.413712936686351895e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.753956198692321777e-01 2.413712936686351895e-04 1.930970320245251060e-04 8.206623606383800507e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.781717061996459961e-01 2.413712936686351895e-04 1.930970320245251060e-04 4.827425800613127649e-05 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.817661643028259277e-01 2.413712936686351895e-04 2.896455407608300447e-04 3.861940640490502119e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.805787205696105957e-01 -3.379197733011096716e-04 4.827425873372703791e-04 -7.723881280981004238e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.781336784362792969e-01 1.062033697962760925e-03 -1.930970320245251060e-04 -1.930970174726098776e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.732047915458679199e-01 -5.310168489813804626e-04 -4.827424709219485521e-05 -1.158582163043320179e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.669371843338012695e-01 -9.654851601226255298e-05 9.654851746745407581e-04 2.896455407608300447e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.658684730529785156e-01 1.448227703804150224e-04 -1.930970320245251060e-04 -1.448227703804150224e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.707965850830078125e-01 -2.413712936686351895e-04 3.861940349452197552e-04 -1.013759407214820385e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.740077257156372070e-01 4.344682965893298388e-04 1.062033697962760925e-03 -5.310168489813804626e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.738549590110778809e-01 -8.689365931786596775e-04 -1.930970320245251060e-04 -5.310168489813804626e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.688839912414550781e-01 3.379197733011096716e-04 -5.310168489813804626e-04 -2.413712645648047328e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.667046070098876953e-01 1.496501849032938480e-03 1.930970320245251060e-04 -2.896455407608300447e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.663263559341430664e-01 0.000000000000000000e+00 5.792910815216600895e-04 1.448227703804150224e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.654842615127563477e-01 1.013759407214820385e-03 6.758395466022193432e-04 2.896455407608300447e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.651207923889160156e-01 -9.172108839266002178e-04 -9.654852328822016716e-05 -5.792910815216600895e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.658937454223632812e-01 2.896455407608300447e-04 8.206623606383800507e-04 -3.379197733011096716e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.657737016677856445e-01 9.172108839266002178e-04 1.013759407214820385e-03 -3.861940640490502119e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.654192328453063965e-01 -8.689365931786596775e-04 -1.930970320245251060e-04 -7.241138373501598835e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.627553820610046387e-01 -6.275653140619397163e-04 -7.723881280981004238e-04 3.379197733011096716e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.562251567840576172e-01 -1.448227994842454791e-04 -9.654851601226255298e-05 1.834421767853200436e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.499741792678833008e-01 -2.896455407608300447e-04 4.827425800613127649e-05 -5.310168489813804626e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.452731013298034668e-01 6.275653140619397163e-04 3.379197733011096716e-04 5.310168489813804626e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.485305547714233398e-01 5.310168489813804626e-04 1.930970349349081516e-03 -1.544776139780879021e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.493297934532165527e-01 8.689366513863205910e-04 1.062033697962760925e-03 -4.344683256931602955e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.442728161811828613e-01 1.930970320245251060e-04 6.758395466022193432e-04 -1.303404918871819973e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.340149760246276855e-01 1.158582163043320179e-03 9.654851746745407581e-04 1.013759407214820385e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.232687354087829590e-01 3.861940349452197552e-04 4.344682674854993820e-04 -4.344682674854993820e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.167028665542602539e-01 2.413712936686351895e-04 2.365438500419259071e-03 -1.737873186357319355e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.126202464103698730e-01 7.241138373501598835e-04 8.689365931786596775e-04 -4.827425873372703791e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.080466032028198242e-01 3.379197733011096716e-04 1.930970320245251060e-04 3.861940349452197552e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.004284739494323730e-01 8.689365349709987640e-04 7.241138373501598835e-04 -7.723881280981004238e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.890992403030395508e-01 2.896455407608300447e-04 -6.275653140619397163e-04 -4.344682674854993820e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.781186342239379883e-01 2.413712936686351895e-04 7.241138373501598835e-04 -1.448227703804150224e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.645519614219665527e-01 2.896455407608300447e-04 4.827425873372703791e-04 4.827425873372703791e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.511860370635986328e-01 9.654850873630493879e-05 1.303404918871819973e-03 2.172341570258140564e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.422345519065856934e-01 -3.861940640490502119e-04 1.930970465764403343e-04 -1.593050314113497734e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.360445499420166016e-01 2.896455407608300447e-04 1.448227703804150224e-04 -1.158582163043320179e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.267869949340820312e-01 2.413712936686351895e-04 -4.827426164411008358e-05 -1.882696058601140976e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.211928009986877441e-01 -1.448227703804150224e-04 5.310168489813804626e-04 4.827426164411008358e-05 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.112432956695556641e-01 -4.827426164411008358e-05 5.792910815216600895e-04 2.413712936686351895e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
7.973561882972717285e-01 9.654851746745407581e-04 1.158582163043320179e-03 -2.365438500419259071e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
7.792838215827941895e-01 9.654851746745407581e-04 6.758395466022193432e-04 -1.930970349349081516e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
7.623451352119445801e-01 -1.930970320245251060e-04 6.758395466022193432e-04 -2.993003698065876961e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
7.485875487327575684e-01 3.861940640490502119e-04 0.000000000000000000e+00 -1.593050430528819561e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
7.319648265838623047e-01 2.896455407608300447e-04 -4.827424709219485521e-05 -2.027518814429640770e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
7.104653120040893555e-01 1.062033697962760925e-03 6.275653140619397163e-04 -4.103311803191900253e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
6.812121868133544922e-01 7.723881280981004238e-04 4.827425873372703791e-04 -6.854944396764039993e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
6.439930200576782227e-01 1.255130628123879433e-03 7.723880698904395103e-04 -8.496269583702087402e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
6.012718081474304199e-01 1.399953383952379227e-03 1.110307872295379639e-03 -9.896221570670604706e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
5.553280115127563477e-01 9.172108839266002178e-04 2.510261256247758865e-03 -1.071688439697027206e-02 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
5.076367855072021484e-01 -5.792910815216600895e-04 1.013759407214820385e-03 -1.037896517664194107e-02 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
4.687365591526031494e-01 -3.861940640490502119e-04 1.448227674700319767e-03 -8.013526909053325653e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
4.439267814159393311e-01 -4.827425873372703791e-04 8.689365931786596775e-04 -5.599813535809516907e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
4.369429945945739746e-01 -2.413712936686351895e-04 7.723881280981004238e-04 -1.399953383952379227e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
4.466319084167480469e-01 -4.827425873372703791e-04 5.310168489813804626e-04 1.786147477105259895e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
4.764082133769989014e-01 6.275653140619397163e-04 1.544776256196200848e-03 5.696361884474754333e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
5.184309482574462891e-01 8.206623606383800507e-04 1.351679093204438686e-03 9.461754001677036285e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
5.649739503860473633e-01 1.255130628123879433e-03 9.654851601226255298e-05 8.206623606383800507e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
6.123837232589721680e-01 9.172108839266002178e-04 -1.206856337375938892e-03 7.048042025417089462e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
6.528376340866088867e-01 8.206623606383800507e-04 4.827425873372703791e-04 6.854944396764039993e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
6.855419874191284180e-01 2.896455407608300447e-04 -4.827425873372703791e-04 6.710121408104896545e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
7.099891304969787598e-01 1.930970320245251060e-04 8.206623606383800507e-04 4.634328652173280716e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
7.311447858810424805e-01 1.255130628123879433e-03 2.896455116569995880e-04 6.661846768110990524e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
7.489495277404785156e-01 7.723881280981004238e-04 -4.827425873372703791e-04 3.668843535706400871e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
7.702373862266540527e-01 3.379197733011096716e-04 -9.654851601226255298e-05 3.668843535706400871e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
7.904498577117919922e-01 -1.448227703804150224e-04 9.654851746745407581e-04 4.441231489181518555e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.036968111991882324e-01 8.689365931786596775e-04 5.792910815216600895e-04 2.268890151754021645e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.143874406814575195e-01 -1.448227994842454791e-04 1.448227558284997940e-04 1.930970349349081516e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.277769684791564941e-01 1.930970320245251060e-04 6.758395466022193432e-04 1.641324721276760101e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.422130942344665527e-01 1.158582163043320179e-03 8.689365931786596775e-04 1.255130628123879433e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.540348410606384277e-01 1.448227791115641594e-03 1.448227703804150224e-04 1.255130628123879433e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.655792474746704102e-01 1.496501849032938480e-03 8.206623024307191372e-04 4.344682965893298388e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.713640570640563965e-01 1.013759407214820385e-03 -1.930970320245251060e-04 1.544776256196200848e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.786691427230834961e-01 1.062033697962760925e-03 1.110307872295379639e-03 1.303404918871819973e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.856825828552246094e-01 8.206623606383800507e-04 1.158582163043320179e-03 8.206623606383800507e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.949548602104187012e-01 5.310168489813804626e-04 6.758395466022193432e-04 6.275653140619397163e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.045252203941345215e-01 5.310168489813804626e-04 2.413712936686351895e-04 4.827430166187696159e-05 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.104104042053222656e-01 1.593050430528819561e-03 1.206856337375938892e-03 -2.896455407608300447e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.115633964538574219e-01 0.000000000000000000e+00 1.206856337375938892e-03 5.310168489813804626e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.154515862464904785e-01 1.930970320245251060e-04 1.351679093204438686e-03 8.206623606383800507e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.236507415771484375e-01 -5.310168489813804626e-04 4.827426164411008358e-05 3.861940640490502119e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.235361814498901367e-01 1.448227703804150224e-04 3.379197733011096716e-04 1.930970320245251060e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.296434521675109863e-01 1.110307872295379639e-03 5.310168489813804626e-04 9.654850873630493879e-05 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.356564879417419434e-01 1.593050314113497734e-03 1.737873186357319355e-03 6.758395466022193432e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.393421411514282227e-01 1.062033697962760925e-03 9.654851601226255298e-05 4.827425873372703791e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.444482922554016113e-01 1.593050430528819561e-03 3.861940640490502119e-04 -7.723881280981004238e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.475056529045104980e-01 1.303404918871819973e-03 3.379197733011096716e-04 1.930970320245251060e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.483556151390075684e-01 4.827425800613127649e-05 -1.930970320245251060e-04 5.310168489813804626e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.436718821525573730e-01 6.758395466022193432e-04 -6.758395466022193432e-04 -1.062033697962760925e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.409719109535217285e-01 1.158582163043320179e-03 6.275653140619397163e-04 4.344682965893298388e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.459953904151916504e-01 0.000000000000000000e+00 -5.310168489813804626e-04 1.013759407214820385e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.516043663024902344e-01 6.758395466022193432e-04 7.723881280981004238e-04 5.792910815216600895e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.550178050994873047e-01 -3.379197733011096716e-04 -7.241138373501598835e-04 1.110307872295379639e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.578662514686584473e-01 1.448227703804150224e-04 1.448227703804150224e-04 4.827424709219485521e-05 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.577305912971496582e-01 -3.861940640490502119e-04 -2.896455407608300447e-04 3.379197733011096716e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.580333828926086426e-01 8.206623606383800507e-04 2.413712936686351895e-04 1.786147477105259895e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.616460204124450684e-01 -2.896455407608300447e-04 -2.413712936686351895e-04 2.896455407608300447e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.689639210700988770e-01 -4.344682674854993820e-04 -1.786147477105259895e-03 4.344682965893298388e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.720373749732971191e-01 7.241138373501598835e-04 -8.206623606383800507e-04 -7.241138373501598835e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.697468876838684082e-01 -1.448227703804150224e-04 -1.062033697962760925e-03 1.062033697962760925e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.687135219573974609e-01 4.827425873372703791e-04 -1.930970174726098776e-04 -4.344682965893298388e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.701699018478393555e-01 -4.344682965893298388e-04 0.000000000000000000e+00 -7.723881280981004238e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.705246090888977051e-01 3.379197733011096716e-04 1.448227703804150224e-04 -9.654851746745407581e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.694918394088745117e-01 0.000000000000000000e+00 -1.930970320245251060e-04 -2.413712645648047328e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.691411852836608887e-01 1.255130628123879433e-03 -1.303404918871819973e-03 6.758395466022193432e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.709924459457397461e-01 1.496501849032938480e-03 -5.310168489813804626e-04 -7.241138373501598835e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.720110893249511719e-01 9.654850873630493879e-05 -9.172108839266002178e-04 1.255130628123879433e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.723017215728759766e-01 9.654851746745407581e-04 8.689366513863205910e-04 1.593050430528819561e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.674819111824035645e-01 7.241138373501598835e-04 1.448227703804150224e-04 -1.930970465764403343e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.621574282646179199e-01 7.723881280981004238e-04 -5.792910815216600895e-04 3.861940349452197552e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.591060280799865723e-01 3.861940349452197552e-04 4.827425873372703791e-04 8.689365931786596775e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.633256196975708008e-01 -1.158582163043320179e-03 -2.896455407608300447e-04 8.689365931786596775e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.703278541564941406e-01 1.110307872295379639e-03 3.379198024049401283e-04 1.255130628123879433e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.741981625556945801e-01 9.654851601226255298e-05 4.827426164411008358e-05 1.979244640097022057e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.722954034805297852e-01 2.413712645648047328e-04 -2.896455407608300447e-04 -3.861940640490502119e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.666289687156677246e-01 2.896455407608300447e-04 5.792910815216600895e-04 1.255130628123879433e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.661362767219543457e-01 -3.861940640490502119e-04 -1.930970174726098776e-04 7.723881280981004238e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.701766967773437500e-01 -1.448227703804150224e-04 -2.896455407608300447e-04 -7.241138373501598835e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.745037555694580078e-01 1.110307872295379639e-03 -1.448227703804150224e-04 1.206856337375938892e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.770098328590393066e-01 -2.896455407608300447e-04 -2.413712936686351895e-04 1.351679093204438686e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.771816134452819824e-01 9.654851601226255298e-05 1.930970320245251060e-04 4.344682965893298388e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.783356189727783203e-01 3.379197441972792149e-04 2.896455407608300447e-04 1.448227674700319767e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.773667454719543457e-01 1.206856337375938892e-03 4.827424709219485521e-05 4.827425873372703791e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.753864407539367676e-01 -9.654850873630493879e-05 -4.827424709219485521e-05 1.351679093204438686e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.701645970344543457e-01 4.827425800613127649e-05 9.654849418438971043e-05 -3.379197733011096716e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.673814177513122559e-01 -4.827425800613127649e-05 1.448227703804150224e-04 2.220615744590759277e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.668898582458496094e-01 0.000000000000000000e+00 8.689365931786596775e-04 -1.593050430528819561e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.718707203865051270e-01 3.379197733011096716e-04 5.310168489813804626e-04 -2.027518814429640770e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.723942875862121582e-01 -3.379197733011096716e-04 5.792910815216600895e-04 -6.758395466022193432e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.669610857963562012e-01 4.827425436815246940e-05 6.275653140619397163e-04 -9.172108839266002178e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.535979032516479492e-01 5.310168489813804626e-04 8.689365931786596775e-04 9.654849418438971043e-05 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.419274330139160156e-01 1.496501965448260307e-03 1.399953383952379227e-03 -9.654851746745407581e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.355358481407165527e-01 -4.344683256931602955e-04 -1.930970320245251060e-04 -1.641324721276760101e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.309619069099426270e-01 7.241138955578207970e-04 1.303404918871819973e-03 2.413712936686351895e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.223014712333679199e-01 4.344682965893298388e-04 1.110307872295379639e-03 -4.827424709219485521e-05 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.070621728897094727e-01 1.399953383952379227e-03 6.758395466022193432e-04 -2.896455407608300447e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.875162601470947266e-01 1.158582163043320179e-03 1.737873186357319355e-03 5.310168489813804626e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.661646842956542969e-01 1.496501849032938480e-03 3.137826453894376755e-03 -1.593050430528819561e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.490344285964965820e-01 1.158582163043320179e-03 1.399953383952379227e-03 1.930970320245251060e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.380950093269348145e-01 1.399953383952379227e-03 2.365438500419259071e-03 -1.303404918871819973e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.308437466621398926e-01 1.110307872295379639e-03 2.317164326086640358e-03 -7.241138373501598835e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.279358744621276855e-01 1.786147477105259895e-03 1.399953383952379227e-03 -4.827425873372703791e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.277686238288879395e-01 3.861940349452197552e-04 1.496501849032938480e-03 3.137826686725020409e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.271431326866149902e-01 3.861940640490502119e-04 1.303404918871819973e-03 5.792910815216600895e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.294001817703247070e-01 5.310168489813804626e-04 1.255130628123879433e-03 1.110307872295379639e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.326122164726257324e-01 7.241138373501598835e-04 2.027518814429640770e-03 8.689365931786596775e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.414869904518127441e-01 -5.792910815216600895e-04 2.268890151754021645e-03 6.758395466022193432e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.587601184844970703e-01 8.206623606383800507e-04 2.510261256247758865e-03 -9.654851746745407581e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.786043524742126465e-01 9.654851601226255298e-05 1.303404918871819973e-03 1.930970349349081516e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
8.959887623786926270e-01 -3.861940640490502119e-04 8.206623606383800507e-04 4.344682965893298388e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.046964049339294434e-01 0.000000000000000000e+00 9.654851746745407581e-04 2.896455407608300447e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.074066281318664551e-01 1.062033697962760925e-03 7.241138373501598835e-04 6.758395466022193432e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.078864455223083496e-01 3.379198024049401283e-04 1.110307872295379639e-03 1.882695942185819149e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.143478870391845703e-01 -8.206623024307191372e-04 -2.413712936686351895e-04 -9.654852328822016716e-05 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.277055859565734863e-01 6.275653140619397163e-04 1.303404918871819973e-03 -5.792910815216600895e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.392797350883483887e-01 1.448227703804150224e-04 -9.654851601226255298e-05 1.110307872295379639e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.450487494468688965e-01 -1.448227994842454791e-04 4.827425800613127649e-05 1.013759407214820385e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.463735222816467285e-01 4.344682674854993820e-04 1.930970320245251060e-04 5.792910815216600895e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.461848139762878418e-01 1.448227703804150224e-04 1.882696058601140976e-03 -9.172108839266002178e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.455249309539794922e-01 6.758395466022193432e-04 4.344683256931602955e-04 4.344682965893298388e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.468216300010681152e-01 -9.654849418438971043e-05 6.275653140619397163e-04 0.000000000000000000e+00 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.537564516067504883e-01 1.448227703804150224e-04 9.172108839266002178e-04 -9.654851601226255298e-05 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.603940248489379883e-01 5.792910815216600895e-04 9.654850873630493879e-05 1.062033697962760925e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.661600589752197266e-01 1.351679093204438686e-03 5.792910815216600895e-04 0.000000000000000000e+00 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.706571698188781738e-01 1.641324721276760101e-03 1.448227674700319767e-03 1.448227703804150224e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.706010818481445312e-01 -2.413712936686351895e-04 1.448227674700319767e-03 -1.930970174726098776e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.645067453384399414e-01 1.641324721276760101e-03 5.310168489813804626e-04 1.255130628123879433e-03 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.567105770111083984e-01 2.413712936686351895e-04 6.275653140619397163e-04 -2.413712936686351895e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.568951725959777832e-01 -4.827427619602531195e-05 9.172108839266002178e-04 -5.310168489813804626e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.636424183845520020e-01 2.413712936686351895e-04 6.275653140619397163e-04 9.654852328822016716e-05 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.704832434654235840e-01 1.641324721276760101e-03 1.158582163043320179e-03 5.310168489813804626e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
9.726838469505310059e-01 2.413712936686351895e-04 1.593050314113497734e-03 0.000000000000000000e+00 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04 7.616745996946300539e-04
So we are now ready for the inversion. Let’s print first the configuration file and then do a simple inversion for a 1D input file. You can see that we are including two atmospheres, a photosphere to explain the Si I line and a chromosphere to explain the He I multiplet. We also give some rough intervals for the parameters.
Let’s also generate a couple of standard atmospheres which will be used as input in the configuration file:
[7]:
tmp = hazel.tools.File_photosphere(mode='single')
tmp.set_default(n_pixel=1, default='hsra')
tmp.save('photospheres/init_spot')
tmp = hazel.tools.File_chromosphere(mode='single')
tmp.set_default(n_pixel=1, default='disk')
tmp.save('chromospheres/init_spot')
Setting HSRA photosphere
Saving photospheric 1D model : photospheres/init_spot.1d
Setting standard chromosphere
Saving chromospheric 1D model : chromospheres/init_spot.1d
[8]:
%cat conf_spot.ini
# Hazel configuration File
[Working mode]
Output file = output.h5
Number of cycles = 2
# Topology
# Always photosphere and then chromosphere
# Photospheres are only allowed to be added with a filling factor
# Atmospheres share a filling factor if they are in parenthesis
# Atmospheres are one after the other with the -> operator
# Atmosphere 1 = ph2 -> ch1 -> ch2
[Spectral regions]
[[Region 1]]
Name = spec1
Topology = ph1 -> ch1
Stokes weights = 1.0, 1.0, 1.0, 1.0
LOS = 0.0, 0.0, 90.0
Boundary condition = 1.0, 0.0, 0.0, 0.0 # I/Ic(mu=1), Q/Ic(mu=1), U/Ic(mu=1), V/Ic(mu=1)
Wavelength file = '10830_spot.wavelength'
Wavelength weight file = '10830_spot.weights'
Observations file = '10830_spot.1d'
Weights Stokes I = 1.0, 0.0, 0.0, 0.0
Weights Stokes Q = 0.0, 10.0, 0.0, 0.0
Weights Stokes U = 0.0, 10.0, 0.0, 0.0
Weights Stokes V = 0.0, 10.0, 0.0, 0.0
Mask file = None
[Atmospheres]
[[Chromosphere 1]]
Name = ch1 # Name of the atmosphere component
Spectral region = spec1 # Spectral region to be used for synthesis
Height = 3.0 # Height of the slab
Line = 10830 # 10830, 5876
Reference atmospheric model = 'chromospheres/init_spot.1d' # File with model parameters
[[[Ranges]]]
Bx = -500, 500
By = -500, 500
Bz = -500, 500
tau = 0.01, 5.0
v = -10.0, 10.0
deltav = 6.0, 12.0
beta = 0.9, 2.0
a = 0.0, 0.1
ff = 0.0, 1.001
[[[Nodes]]]
Bx = 0, 1
By = 0, 1
Bz = 0, 1
tau = 1, 0
v = 1, 0
deltav = 1, 0
beta = 0, 0
a = 1, 0
ff = 0, 0
[[Photosphere 1]]
Name = ph1
Reference atmospheric model = 'photospheres/init_spot.1d'
Spectral region = spec1
Spectral lines = 300,
[[[Ranges]]]
T = 0.0, 10000.0
vmic = 0.0, 3.0
v = -10.0, 10.0
Bx = -1000.0, 1000.0
By = -1000.0, 1000.0
Bz = -1000.0, 1000.0
ff = 0, 1.00
[[[Nodes]]]
T = 3, 0
vmic = 1, 0
v = 1, 0
Bx = 0, 0
By = 0, 0
Bz = 0, 1
ff = 0, 0
[9]:
mod = hazel.Model('conf_spot.ini', working_mode='inversion', verbose=3)
mod.read_observation()
mod.open_output()
mod.invert()
mod.write_output()
mod.close_output()
2018-10-24 09:53:51,511 - Using configuration from file : conf_spot.ini
2018-10-24 09:53:51,515 - Adding spectral region spec1
2018-10-24 09:53:51,516 - - Reading wavelength axis from 10830_spot.wavelength
2018-10-24 09:53:51,521 - - Reading wavelength weights from 10830_spot.weights
2018-10-24 09:53:51,526 - - Using observations from 10830_spot.1d
2018-10-24 09:53:51,527 - - No mask for pixels
2018-10-24 09:53:51,528 - - No instrumental profile
2018-10-24 09:53:51,529 - - Using LOS ['0.0', '0.0', '90.0']
2018-10-24 09:53:51,530 - - Using boundary condition ['1.0', '0.0', '0.0', '0.0']
2018-10-24 09:53:51,531 - Using 2 cycles
2018-10-24 09:53:51,532 - Not using randomizations
2018-10-24 09:53:51,533 - Adding atmospheres
2018-10-24 09:53:51,533 - - New available chromosphere : ch1
2018-10-24 09:53:51,534 - * Adding line : 10830
2018-10-24 09:53:51,535 - * Magnetic field reference frame : vertical
2018-10-24 09:53:51,536 - * Reading 1D model chromospheres/init_spot.1d as reference
2018-10-24 09:53:51,540 - - New available photosphere : ph1
2018-10-24 09:53:51,542 - * Adding line : [300]
2018-10-24 09:53:51,542 - * Magnetic field reference frame : vertical
2018-10-24 09:53:51,544 - * Reading 1D model photospheres/init_spot.1d as reference
2018-10-24 09:53:51,547 - Adding topologies
2018-10-24 09:53:51,547 - - ph1 -> ch1
2018-10-24 09:53:51,548 - Removing unused atmospheres
2018-10-24 09:53:51,550 - Number of pixels to invert : 1
2018-10-24 09:53:51,554 - Total number of free parameters in all cycles : 13
2018-10-24 09:53:51,600 - -------------
2018-10-24 09:53:51,601 - Cycle 0
2018-10-24 09:53:51,601 - Weights for region spec1 : SI=1.0 - SQ=0.0 - SU=0.0 - SV=0.0
2018-10-24 09:53:51,602 - -------------
2018-10-24 09:53:51,603 - Free parameters for ch1
2018-10-24 09:53:51,604 - - tau with 1 node
2018-10-24 09:53:51,605 - - v with 1 node
2018-10-24 09:53:51,606 - - deltav with 1 node
2018-10-24 09:53:51,607 - - a with 1 node
2018-10-24 09:53:51,607 - Free parameters for ph1
2018-10-24 09:53:51,608 - - T with 3 nodes
2018-10-24 09:53:51,609 - - vmic with 1 node
2018-10-24 09:53:51,610 - - v with 1 node
/scratch/Dropbox/GIT/hazel2/hazel/transforms.py:21: RuntimeWarning: divide by zero encountered in double_scalars
return np.log(x / (1.0 - x))
2018-10-24 09:53:53,707 - * Optimal lambda: 0.009999999999999997
2018-10-24 09:53:54,311 - It: 0 - chi2: 730.047874343801 - lambda: 0.009999999999999997 - rel: -1.9999997079808716
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.275 -1.514 10.214 1.000 0.000
2018-10-24 09:53:55,248 - * Optimal lambda: 0.0031329840216438656
2018-10-24 09:53:55,856 - It: 1 - chi2: 219.67708243514014 - lambda: 0.0031329840216438656 - rel: -1.0747759933352057
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.241 -4.468 11.571 1.000 0.000
2018-10-24 09:53:56,653 - * Optimal lambda: 0.005397943999573633
2018-10-24 09:53:57,300 - It: 2 - chi2: 88.44700424554424 - lambda: 0.005397943999573633 - rel: -0.8518001925996291
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.290 -4.669 9.299 1.000 0.000
2018-10-24 09:53:58,804 - * Optimal lambda: 0.000539794399957363
2018-10-24 09:53:59,463 - It: 3 - chi2: 62.940986344325864 - lambda: 0.000539794399957363 - rel: -0.33696223593214225
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.292 -4.969 9.963 1.000 0.000
2018-10-24 09:54:00,704 - * Optimal lambda: 0.0005397943999573629
2018-10-24 09:54:01,353 - It: 4 - chi2: 62.366510912066666 - lambda: 0.0005397943999573629 - rel: -0.009169051251319154
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.291 -4.884 10.037 1.000 0.000
2018-10-24 09:54:02,065 - * Optimal lambda: 0.0020434336502263206
2018-10-24 09:54:02,718 - It: 5 - chi2: 62.34719752764511 - lambda: 0.0020434336502263206 - rel: -0.00030972352058465804
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.291 -4.873 10.077 1.000 0.000
2018-10-24 09:54:04,091 - * Optimal lambda: 6.461904582147022
2018-10-24 09:54:04,627 - It: 6 - chi2: 62.34706661811411 - lambda: 6.461904582147022 - rel: -2.0996880953866914e-06
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.291 -4.872 10.078 1.000 0.000
2018-10-24 09:54:05,174 - -------------
2018-10-24 09:54:05,175 - Cycle 1
2018-10-24 09:54:05,176 - Weights for region spec1 : SI=0.0 - SQ=10.0 - SU=10.0 - SV=10.0
2018-10-24 09:54:05,176 - -------------
2018-10-24 09:54:05,177 - Free parameters for ch1
2018-10-24 09:54:05,178 - - Bx with 1 node
2018-10-24 09:54:05,179 - - By with 1 node
2018-10-24 09:54:05,180 - - Bz with 1 node
2018-10-24 09:54:05,181 - Free parameters for ph1
2018-10-24 09:54:05,182 - - Bz with 1 node
2018-10-24 09:54:07,470 - * Optimal lambda: 0.6461904582147021
2018-10-24 09:54:08,172 - It: 0 - chi2: 16.730145131714785 - lambda: 0.6461904582147021 - rel: -1.153731156599563
Bx By Bz tau v deltav beta a
-0.014 0.014 -22.413 0.291 -4.872 10.078 1.000 0.000
2018-10-24 09:54:09,330 - * Optimal lambda: 0.26610281324782875
2018-10-24 09:54:10,033 - It: 1 - chi2: 13.42091457596078 - lambda: 0.26610281324782875 - rel: -0.21951006616935417
Bx By Bz tau v deltav beta a
216.671 -499.982 -32.376 0.291 -4.872 10.078 1.000 0.000
2018-10-24 09:54:10,886 - * Optimal lambda: 0.7340985960149292
2018-10-24 09:54:11,585 - It: 2 - chi2: 15.868097531285427 - lambda: 0.7340985960149292 - rel: 0.16710587208362726
Bx By Bz tau v deltav beta a
255.481 500.000 -33.334 0.291 -4.872 10.078 1.000 0.000
2018-10-24 09:54:12,249 - * Optimal lambda: 9.907551591772469
2018-10-24 09:54:12,954 - It: 3 - chi2: 15.208039400657768 - lambda: 9.907551591772469 - rel: -0.042480063212051604
Bx By Bz tau v deltav beta a
256.706 500.000 -37.089 0.291 -4.872 10.078 1.000 0.000
2018-10-24 09:54:13,451 - * Optimal lambda: 320.00667607585524
2018-10-24 09:54:14,157 - It: 4 - chi2: 15.206620836057217 - lambda: 320.00667607585524 - rel: -9.328163389035902e-05
Bx By Bz tau v deltav beta a
256.686 500.000 -37.035 0.291 -4.872 10.078 1.000 0.000
NWe see that we found a solution with a relatively good \(\chi^2\) and now let’s analyze the results. For your specific case, you probably need some trial and error on the Stokes weights and range of parameters to find a reliable solution.
[10]:
f = h5py.File('output.h5', 'r')
print('(npix,nrand,ncycle,nstokes,nlambda) -> {0}'.format(f['spec1']['stokes'].shape))
fig, ax = pl.subplots(nrows=2, ncols=2, figsize=(10,10))
ax = ax.flatten()
for i in range(4):
ax[i].plot(f['spec1']['wavelength'][:] - 10830, stokes[i,:])
for j in range(2):
ax[i].plot(f['spec1']['wavelength'][:] - 10830, f['spec1']['stokes'][0,0,j,i,:])
for i in range(4):
ax[i].set_xlabel('Wavelength - 10830[$\AA$]')
ax[i].set_ylabel('{0}/Ic'.format(label[i]))
ax[i].set_xlim([-7,3])
#pl.tight_layout()
f.close()
(npix,nrand,ncycle,nstokes,nlambda) -> (1, 1, 2, 4, 210)
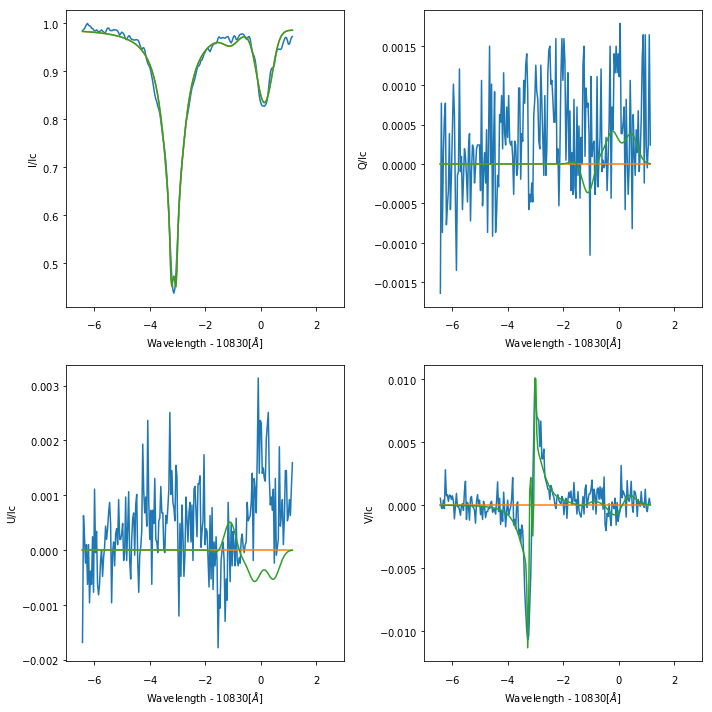
Now the photospheric model:
[11]:
f = h5py.File('output.h5', 'r')
print('(npix,nrand,ncycle,nz) -> {0}'.format(f['ph1']['T'].shape))
fig, ax = pl.subplots(nrows=1, ncols=3, figsize=(10,5))
ax = ax.flatten()
for i in range(2):
ax[0].plot(f['ph1']['log_tau'][:], f['ph1']['T'][0,0,i,:])
for i in range(2):
ax[1].plot(f['ph1']['log_tau'][:], f['ph1']['v'][0,0,i,:])
for i in range(2):
ax[2].plot(f['ph1']['log_tau'][:], f['ph1']['Bz'][0,0,i,:])
ax[0].set_xlabel(r'$\tau_{500}$')
ax[0].set_ylabel('T [K]')
ax[1].set_xlabel(r'$\tau_{500}$')
ax[1].set_ylabel('v [km/s]')
ax[2].set_xlabel(r'$\tau_{500}$')
ax[2].set_ylabel('Bz [G]')
#pl.tight_layout()
f.close()
(npix,nrand,ncycle,nz) -> (1, 1, 2, 73)
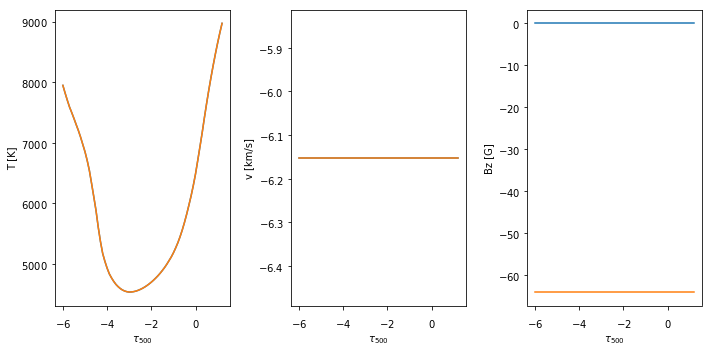
6.3.2. Spicules¶
Let’s try to invert observations from spicules. First, we read the observations from the datafile (already on the correct reference system and with the wavelength calibration).
[12]:
tmp = io.readsav('/scratch/Dropbox/test/test_hazel2/orozco/Spiculas_map.sav')
print(tmp.keys())
print(tmp['heperf'].shape)
f, ax = pl.subplots(nrows=1, ncols=2, figsize=(10,6))
ax[0].imshow(tmp['heperf'][:,0,:,0])
ax[1].imshow(tmp['heperf'][:,0,:,100])
f, ax = pl.subplots(nrows=2, ncols=2, figsize=(10,10))
stokes = np.zeros((4,101))
stokes[0,:] = tmp['heperf'][10,0,175,:] / np.max(tmp['heperf'][10,0,175,:])
stokes[1,:] = tmp['heperf'][10,1,175,:] / np.max(tmp['heperf'][10,0,175,:])
stokes[2,:] = tmp['heperf'][10,2,175,:] / np.max(tmp['heperf'][10,0,175,:])
stokes[3,:] = tmp['heperf'][10,3,175,:] / np.max(tmp['heperf'][10,0,175,:])
ax[0,0].plot(tmp['lambda'], stokes[0,:])
ax[0,1].plot(tmp['lambda'], stokes[1,:])
ax[1,0].plot(tmp['lambda'], stokes[2,:])
ax[1,1].plot(tmp['lambda'], stokes[3,:])
n_lambda = len(tmp['lambda'])
dict_keys(['lambda', 'heperf', 'mask_heights'])
(25, 4, 224, 101)

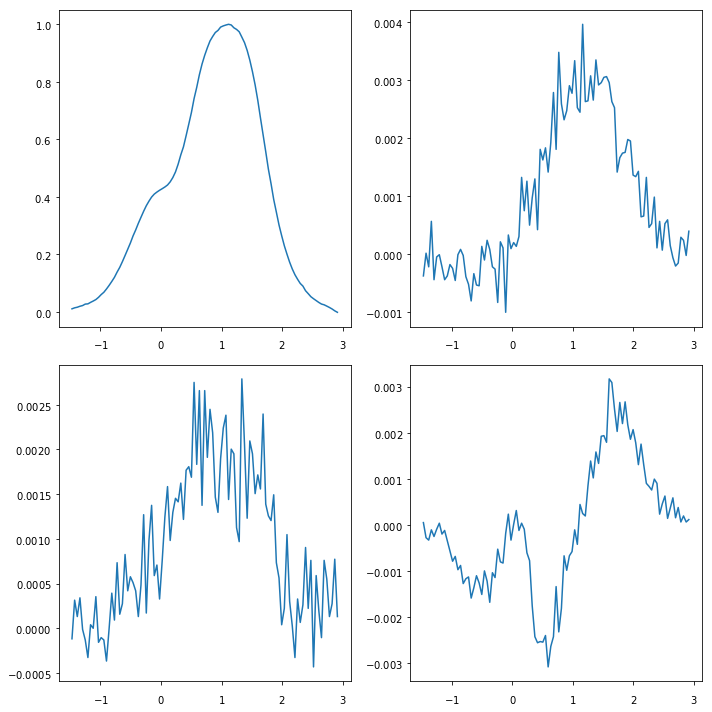
The first thing we do is estimate the noise standard deviation. You should do it more carefully so that the final values of the \(\chi^2\) are close to one in case the observed profile can be correctly represented with the simple Hazel atmosphere. For the moment and as a quick estimation we just estimate it via the standard deviation in a small region close to the continuum.
[13]:
noise=np.std(stokes[1,0:15])
print(noise)
0.00026189987900385165
Then we save several files with the observations. The first one the file with the wavelength axis. Remember that this axis is common to all observed pixels in case HDF5 files are used. We add 10829.0911 Angstrom because the osberved files are referred to this wavelength (it is the center of the multiplet).
[14]:
f = hazel.tools.File_observation(mode='single')
f.set_size(n_lambda=n_lambda, n_pixel=1)
print(f.obs['stokes'].shape)
f.obs['wavelength'] = tmp['lambda']+10829.0911
f.obs['stokes'][0,:,:] = stokes.T
f.obs['sigma'] = noise*np.ones((n_lambda,4))
f.obs['los'][0,:] = np.array([90.0,0.0,90.0])
f.obs['boundary'][:] = 0.0
f.obs['weights'][:] = 1.0
f.save('10830_spicule')
(1, 101, 4)
Saving wavelength file : 10830_spicule.wavelength
Saving weights file : 10830_spicule.weights
Saving 1D Stokes file : 10830_spicule.1d
[15]:
%cat 10830_spicule.1d
# LOS theta_LOS, phi_LOS, gamma_LOS
90.0 0.0 90.0
# Boundary condition I/Ic(mu=1), Q/Ic(mu=1), U/Ic(mu=1), V/Ic(mu=1)
0.0 0.0 0.0 0.0
# SI SQ SU SV sigmaI sigmaQ sigmaU sigmaV
1.065849605947732925e-02 -3.797253011725842953e-04 -1.178457823698408902e-04 5.237590085016563535e-05 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.414149347692728043e-02 1.309397521254140884e-05 3.142554196529090405e-04 -2.749734849203377962e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.621034182608127594e-02 -2.225975767942145467e-04 1.309397484874352813e-04 -3.273493784945458174e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.961477473378181458e-02 5.630409577861428261e-04 3.404433664400130510e-04 -1.047518017003312707e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
2.199787832796573639e-02 -4.451951535884290934e-04 -1.309397521254140884e-05 -2.487855381332337856e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
2.710452862083911896e-02 -5.237590085016563535e-05 -1.309397484874352813e-04 -9.165782830677926540e-05 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
2.792944945394992828e-02 -1.309397521254140884e-05 -3.273493784945458174e-04 3.928192745661363006e-05 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
3.307538107037544250e-02 -2.225975767942145467e-04 3.928192745661363006e-05 -1.964096300071105361e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
3.772374242544174194e-02 -4.451951535884290934e-04 0.000000000000000000e+00 -1.178457823698408902e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
4.285658150911331177e-02 -3.797253011725842953e-04 3.535373252816498280e-04 -3.404433664400130510e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
5.060821399092674255e-02 -1.833156566135585308e-04 -1.571277098264545202e-04 -5.630409577861428261e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
6.012753397226333618e-02 -2.487855381332337856e-04 -1.047518017003312707e-04 -7.856385200284421444e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
6.815414130687713623e-02 -4.582891415338963270e-04 -1.309397484874352813e-04 -6.808867328800261021e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
7.963755726814270020e-02 -1.309397521254140884e-05 -3.666313132271170616e-04 -9.689541766420006752e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.226015210151672363e-02 7.856385491322726011e-05 0.000000000000000000e+00 -8.772963774390518665e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.058647930622100830e-01 -2.618795042508281767e-05 3.928192600142210722e-04 -1.270115608349442482e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.202419772744178772e-01 -3.928192600142210722e-04 9.165782830677926540e-05 -1.165363821201026440e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.383771300315856934e-01 -5.237589939497411251e-04 7.332626264542341232e-04 -1.126081915572285652e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.549017280340194702e-01 -8.118264959193766117e-04 1.571277098264545202e-04 -1.584371086210012436e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.747914850711822510e-01 -3.404433664400130510e-04 2.749734849203377962e-04 -1.361773465760052204e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.960691958665847778e-01 -5.368529818952083588e-04 8.249204256571829319e-04 -1.099893939681351185e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
2.177004367113113403e-01 -5.499469698406755924e-04 4.190072068013250828e-04 -1.257021678611636162e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
2.394495308399200439e-01 1.309397484874352813e-04 5.761349457316100597e-04 -1.505807158537209034e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
2.639483511447906494e-01 -1.047518017003312707e-04 5.106650642119348049e-04 -9.951421525329351425e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
2.848856151103973389e-01 2.356915647396817803e-04 4.190072068013250828e-04 -1.217739772982895374e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
3.079441189765930176e-01 7.856385491322726011e-05 1.309397484874352813e-04 -1.676028827205300331e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
3.290123343467712402e-01 -2.225975767942145467e-04 4.844770883210003376e-04 -1.034424058161675930e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
3.499364852905273438e-01 -2.618794969748705626e-04 1.270115608349442482e-03 -1.139175845310091972e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
3.690798878669738770e-01 -8.380144136026501656e-04 1.702216832200065255e-04 -5.237589939497411251e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
3.850152492523193359e-01 2.095036034006625414e-04 9.820481063798069954e-04 -7.987325079739093781e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
3.992877006530761719e-01 1.047518017003312707e-04 1.374867395497858524e-03 -8.249204256571829319e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
4.095533788204193115e-01 -1.008236082270741463e-03 5.892288754694163799e-04 -1.833156566135585308e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
4.166502952575683594e-01 3.273493784945458174e-04 7.070746505632996559e-04 2.356915647396817803e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
4.229092299938201904e-01 9.165782830677926540e-05 3.273493784945458174e-04 -3.273493784945458174e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
4.284217953681945801e-01 1.964096300071105361e-04 7.594506023451685905e-04 1.309397521254140884e-05 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
4.342878758907318115e-01 1.309397484874352813e-04 1.243927632458508015e-03 3.142554196529090405e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
4.412407875061035156e-01 3.011614317074418068e-04 1.584371086210012436e-03 -1.178457823698408902e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
4.521873593330383301e-01 1.322491560131311417e-03 9.820481063798069954e-04 3.928192745661363006e-05 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
4.671668708324432373e-01 7.463566143997013569e-04 1.296303584240376949e-03 -9.165782830677926540e-05 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
4.867423474788665771e-01 1.257021678611636162e-03 1.453431323170661926e-03 -6.023228634148836136e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
5.137421488761901855e-01 4.975710762664675713e-04 1.414149301126599312e-03 -7.725445320829749107e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
5.460842251777648926e-01 9.689541766420006752e-04 1.623652991838753223e-03 -1.754592754878103733e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
5.740005970001220703e-01 1.296303584240376949e-03 1.217739772982895374e-03 -2.422385383397340775e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
6.127064228057861328e-01 4.190072068013250828e-04 1.767686684615910053e-03 -2.553325146436691284e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
6.527346968650817871e-01 1.806968590244650841e-03 1.806968590244650841e-03 -2.527137286961078644e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
6.933783888816833496e-01 1.623652991838753223e-03 1.689122873358428478e-03 -2.540231216698884964e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
7.416689395904541016e-01 1.833156566135585308e-03 2.749734790995717049e-03 -2.396197523921728134e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
7.808985114097595215e-01 1.414149301126599312e-03 1.833156566135585308e-03 -3.077084198594093323e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
8.248419165611267090e-01 1.924814423546195030e-03 2.658077050000429153e-03 -2.631888957694172859e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
8.617014288902282715e-01 2.789016813039779663e-03 1.374867395497858524e-03 -2.422385383397340775e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
8.921318054199218750e-01 1.806968590244650841e-03 2.658077050000429153e-03 -1.335585489869117737e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.183721542358398438e-01 3.482997417449951172e-03 1.911720377393066883e-03 -2.317633712664246559e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.420329928398132324e-01 2.592607168480753899e-03 2.448573475703597069e-03 -1.806968590244650841e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.576017260551452637e-01 2.317633712664246559e-03 2.186693949624896049e-03 -6.677927449345588684e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.714813232421875000e-01 2.474761335179209709e-03 1.466525252908468246e-03 -9.820481063798069954e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.786829948425292969e-01 2.906862646341323853e-03 1.296303584240376949e-03 -6.677927449345588684e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.902711510658264160e-01 2.775922883301973343e-03 1.898626447655260563e-03 -5.761349457316100597e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.944612383842468262e-01 3.338963724672794342e-03 2.239069901406764984e-03 -1.047518017003312707e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.975121617317199707e-01 2.527137286961078644e-03 2.383103594183921814e-03 -4.190072068013250828e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.000000000000000000e+00 2.448573475703597069e-03 1.440337277017533779e-03 4.451951535884290934e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.976300001144409180e-01 3.967474680393934250e-03 2.003378234803676605e-03 2.487855381332337856e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.878880977630615234e-01 2.631888957694172859e-03 1.951002399437129498e-03 1.964096300071105361e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.822314977645874023e-01 2.644983120262622833e-03 1.126081915572285652e-03 8.511084015481173992e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.739953875541687012e-01 3.077084198594093323e-03 9.689541766420006752e-04 1.387961441650986671e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.554018974304199219e-01 2.658077050000429153e-03 2.789016813039779663e-03 1.021330128423869610e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.362585544586181641e-01 3.352057654410600662e-03 2.055754186585545540e-03 1.584371086210012436e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.093242287635803223e-01 2.919956576079130173e-03 1.230833702720701694e-03 1.335585489869117737e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
8.753584623336791992e-01 2.959238365292549133e-03 2.095035975798964500e-03 1.924814423546195030e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
8.350813984870910645e-01 3.050896339118480682e-03 1.951002399437129498e-03 1.937908353284001350e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
7.892917394638061523e-01 3.063990268856287003e-03 1.505807158537209034e-03 1.793874660506844521e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
7.358683347702026367e-01 2.959238365292549133e-03 1.715310732834041119e-03 3.168742172420024872e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
6.749944090843200684e-01 2.631888957694172859e-03 1.558183110319077969e-03 3.090178128331899643e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
6.160322427749633789e-01 2.527137286961078644e-03 2.396197523921728134e-03 2.514043357223272324e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
5.570963025093078613e-01 1.414149301126599312e-03 1.387961441650986671e-03 2.029566094279289246e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
4.961830973625183105e-01 1.662934897467494011e-03 1.257021678611636162e-03 2.658077050000429153e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
4.457843899726867676e-01 1.741498708724975586e-03 1.204645726829767227e-03 2.199787879362702370e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
3.909730017185211182e-01 1.754592754878103733e-03 1.492713228799402714e-03 2.671170979738235474e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
3.468201160430908203e-01 1.977190375328063965e-03 7.332626264542341232e-04 2.173600019887089729e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
3.021172881126403809e-01 1.951002399437129498e-03 5.630409577861428261e-04 1.859344542026519775e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
2.650220692157745361e-01 1.361773465760052204e-03 3.928192745661363006e-05 2.068848116323351860e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
2.301004379987716675e-01 1.335585489869117737e-03 2.225975767942145467e-04 1.780780614353716373e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
2.006520777940750122e-01 1.427243347279727459e-03 1.047517987899482250e-03 1.309397513978183270e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.729190349578857422e-01 6.416047690436244011e-04 3.011614317074418068e-04 1.754592754878103733e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.491927504539489746e-01 6.546987569890916348e-04 2.618795042508281767e-05 1.309397513978183270e-03 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.292637288570404053e-01 1.322491560131311417e-03 -3.273493784945458174e-04 9.034842951223254204e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.133676394820213318e-01 4.582891415338963270e-04 3.273493784945458174e-04 8.380144136026501656e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
9.888570010662078857e-02 5.237589939497411251e-04 6.546987424371764064e-05 7.594506023451685905e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
8.974611014127731323e-02 9.820481063798069954e-04 2.618794969748705626e-04 9.951421525329351425e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
7.356195151805877686e-02 1.047518017003312707e-04 9.034842951223254204e-04 9.034842951223254204e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
6.342721730470657349e-02 5.630409577861428261e-04 2.225975767942145467e-04 2.356915647396817803e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
5.286037921905517578e-02 6.546987424371764064e-05 7.594506023451685905e-04 4.582891415338963270e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
4.578963294625282288e-02 5.237589939497411251e-04 -4.321011947467923164e-04 6.285108393058180809e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
3.929502144455909729e-02 5.892288754694163799e-04 5.892288754694163799e-04 1.440337364329025149e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
3.281350433826446533e-02 1.440337364329025149e-04 1.964096300071105361e-04 3.666313132271170616e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
2.724856324493885040e-02 -6.546987424371764064e-05 -1.047518017003312707e-04 5.892288754694163799e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
2.462976798415184021e-02 -2.095036034006625414e-04 7.594506023451685905e-04 1.571277098264545202e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
2.008615806698799133e-02 -1.571277098264545202e-04 5.499469698406755924e-04 3.797253011725842953e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.538542099297046661e-02 2.880674728658050299e-04 1.309397484874352813e-04 6.546987424371764064e-05 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
1.008236128836870193e-02 2.356915647396817803e-04 2.749734849203377962e-04 1.964096300071105361e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
3.666313132271170616e-03 -2.618795042508281767e-05 7.725445320829749107e-04 6.546987424371764064e-05 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
-1.427243347279727459e-03 3.928192600142210722e-04 1.309397484874352813e-04 1.178457823698408902e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04 2.618998790038516472e-04
We print now the configuration file used in this case. Note that we do three cycles. The first one is used to estimate thermodynamical parameters from Stokes \(I\). The second one is used to get information from the LOS component of the magnetic field using only Stokes \(V\) (in this case the LOS lines along the X axis because \(\theta=90\deg\) and \(\chi=0\deg\)). Finally, the third cycle gets information from Stokes \(Q\) and \(U\) to fix the other two components of the magnetic field. Some trial-and-error is necessary for setting up the weights. Also note that we fix the height to 3’’. One should measure this number better in the observations and set it appropriately.
[16]:
%cat conf_spicules.ini
# Hazel configuration File
[Working mode]
Output file = output.h5
Number of cycles = 3
# Topology
# Always photosphere and then chromosphere
# Photospheres are only allowed to be added with a filling factor
# Atmospheres share a filling factor if they are in parenthesis
# Atmospheres are one after the other with the -> operator
# Atmosphere 1 = ph2 -> ch1 -> ch2
[Spectral regions]
[[Region 1]]
Name = spec1
#Wavelength = 10826, 10833, 150
Topology = ch1
Stokes weights = 1.0, 1.0, 1.0, 1.0
LOS = 90.0, 0.0, 90.0
Boundary condition = 0.0, 0.0, 0.0, 0.0 # I/Ic(mu=1), Q/Ic(mu=1), U/Ic(mu=1), V/Ic(mu=1)
Wavelength file = '10830_spicule.wavelength'
Wavelength weight file = '10830_spicule.weights'
Observations file = '10830_spicule.1d'
Weights Stokes I = 1.0, 0.1, 0.1, 0.0
Weights Stokes Q = 0.0, 0.0, 1.0, 0.0
Weights Stokes U = 0.0, 0.0, 1.0, 0.0
Weights Stokes V = 0.0, 1.0, 1.0, 0.0
Mask file = None
[Atmospheres]
[[Chromosphere 1]]
Name = ch1 # Name of the atmosphere component
Spectral region = spec1 # Spectral region to be used for synthesis
Height = 3.0 # Height of the slab
Line = 10830 # 10830, 5876
Wavelength = 10826, 10833 # Wavelength range used for synthesis
Reference atmospheric model = 'chromospheres/init_spicule.1d' # File with model parameters
[[[Ranges]]]
Bx = -500, 500
By = -500, 500
Bz = -1000, 1000
tau = 0.01, 5.0
v = -10.0, 10.0
deltav = 6.0, 20.0
beta = 0.9, 2.0
a = 0.0, 0.1
ff = 0.0, 1.001
[[[Nodes]]]
Bx = 0, 1, 0
By = 0, 0, 1
Bz = 0, 0, 1
tau = 1, 0, 0
v = 1, 0, 0
deltav = 1, 0, 0
beta = 0, 0, 0
a = 1, 0, 0
ff = 0, 0, 0
Let’s generate an initial model more appropriate for off-limb observations:
[17]:
tmp = hazel.tools.File_chromosphere(mode='single')
tmp.set_default(n_pixel=1, default='offlimb')
tmp.save('chromospheres/init_spicule')
Setting standard chromosphere
Saving chromospheric 1D model : chromospheres/init_spicule.1d
Finally, let’s carry out the inversion. We activate some verbosity to analyze the inversion.
[20]:
mod = hazel.Model('conf_spicules.ini', working_mode='inversion', verbose=2)
mod.read_observation()
mod.open_output()
mod.invert()
mod.write_output()
mod.close_output()
2018-10-24 09:57:09,923 - Using configuration from file : conf_spicules.ini
2018-10-24 09:57:09,925 - Adding spectral region spec1
2018-10-24 09:57:09,926 - - Reading wavelength axis from 10830_spicule.wavelength
2018-10-24 09:57:09,928 - - Reading wavelength weights from 10830_spicule.weights
2018-10-24 09:57:09,930 - - Using observations from 10830_spicule.1d
2018-10-24 09:57:09,931 - - No mask for pixels
2018-10-24 09:57:09,932 - - No instrumental profile
2018-10-24 09:57:09,933 - - Using LOS ['90.0', '0.0', '90.0']
2018-10-24 09:57:09,934 - - Using off-limb normalization (peak intensity)
2018-10-24 09:57:09,935 - - Using boundary condition ['0.0', '0.0', '0.0', '0.0']
2018-10-24 09:57:09,936 - Using 3 cycles
2018-10-24 09:57:09,937 - Not using randomizations
2018-10-24 09:57:09,937 - Adding atmospheres
2018-10-24 09:57:09,938 - - New available chromosphere : ch1
2018-10-24 09:57:09,938 - * Adding line : 10830
2018-10-24 09:57:09,939 - * Magnetic field reference frame : vertical
2018-10-24 09:57:09,941 - * Reading 1D model chromospheres/init_spicule.1d as reference
2018-10-24 09:57:09,943 - Adding topologies
2018-10-24 09:57:09,944 - - ch1
2018-10-24 09:57:09,945 - Removing unused atmospheres
2018-10-24 09:57:09,946 - Number of pixels to invert : 1
2018-10-24 09:57:09,947 - Total number of free parameters in all cycles : 7
2018-10-24 09:57:09,972 - -------------
2018-10-24 09:57:09,973 - Cycle 0
2018-10-24 09:57:09,974 - Weights for region spec1 : SI=1.0 - SQ=0.0 - SU=0.0 - SV=0.0
2018-10-24 09:57:09,975 - -------------
2018-10-24 09:57:11,231 - It: 0 - chi2: 9864.266121175833 - lambda: 0.36942931526143663 - rel: -1.9999960542974438
2018-10-24 09:57:12,541 - It: 1 - chi2: 1126.513253861626 - lambda: 0.03694293152614364 - rel: -1.5900151516387668
2018-10-24 09:57:13,789 - It: 2 - chi2: 948.7208431711406 - lambda: 0.003694293152614363 - rel: -0.17134684799628
2018-10-24 09:57:15,027 - It: 3 - chi2: 941.9987580792599 - lambda: 0.0003694293152614362 - rel: -0.0071106102538262324
2018-10-24 09:57:16,088 - It: 4 - chi2: 941.8592759931872 - lambda: 0.0003694293152614361 - rel: -0.00014808131350658402
2018-10-24 09:57:16,658 - It: 5 - chi2: 941.8554540472019 - lambda: 0.01646928095216359 - rel: -4.057881933395348e-06
2018-10-24 09:57:16,910 - -------------
2018-10-24 09:57:16,911 - Cycle 1
2018-10-24 09:57:16,912 - Weights for region spec1 : SI=0.1 - SQ=0.0 - SU=0.0 - SV=1.0
2018-10-24 09:57:16,913 - -------------
2018-10-24 09:57:18,050 - It: 0 - chi2: 102.20501762215777 - lambda: 1.646928095216359 - rel: -1.6084325749494526
2018-10-24 09:57:19,106 - It: 1 - chi2: 96.02910658777319 - lambda: 0.1646928095216358 - rel: -0.06230926243399206
2018-10-24 09:57:20,166 - It: 2 - chi2: 95.96053131771023 - lambda: 0.01646928095216358 - rel: -0.0007143642835214925
2018-10-24 09:57:20,572 - It: 3 - chi2: 95.96042406896731 - lambda: 0.30396343630250444 - rel: -1.1176345251632026e-06
2018-10-24 09:57:20,740 - -------------
2018-10-24 09:57:20,741 - Cycle 2
2018-10-24 09:57:20,742 - Weights for region spec1 : SI=0.1 - SQ=5.0 - SU=1.0 - SV=1.0
2018-10-24 09:57:20,743 - -------------
2018-10-24 09:57:22,052 - It: 0 - chi2: 137.29018367293264 - lambda: 961.2167841274243 - rel: 0.3543807238410128
2018-10-24 09:57:23,201 - It: 1 - chi2: 136.16971823193478 - lambda: 96.12167841274238 - rel: -0.008194732998826677
2018-10-24 09:57:24,342 - It: 2 - chi2: 127.45373835648553 - lambda: 9.612167841274236 - rel: -0.06612446394750823
2018-10-24 09:57:25,490 - It: 3 - chi2: 112.40224465317975 - lambda: 0.9612167841274232 - rel: -0.12550442573449805
2018-10-24 09:57:26,629 - It: 4 - chi2: 103.85952326796692 - lambda: 0.09612167841274227 - rel: -0.07900352861563284
2018-10-24 09:57:27,368 - It: 5 - chi2: 103.75220994768458 - lambda: 0.057553378091725414 - rel: -0.0010337885881514719
2018-10-24 09:57:28,183 - It: 6 - chi2: 103.74870807189373 - lambda: 0.012620778868004143 - rel: -3.375287034166886e-05
After some seconds, we end up with the following fit for all cycles:
[21]:
f = h5py.File('output.h5', 'r')
print('(npix,nrand,ncycle,nstokes,nlambda) -> {0}'.format(f['spec1']['stokes'].shape))
ncycle = f['spec1']['stokes'].shape[2]
fig, ax = pl.subplots(nrows=2, ncols=2, figsize=(10,10))
ax = ax.flatten()
for i in range(4):
ax[i].plot(f['spec1']['wavelength'][:] - 10830, stokes[i,:])
for j in range(ncycle):
ax[i].plot(f['spec1']['wavelength'][:] - 10830, f['spec1']['stokes'][0,0,j,i,:], label='Cycle {0}'.format(j))
for i in range(4):
ax[i].set_xlabel('Wavelength - 10830[$\AA$]')
ax[i].set_ylabel('{0}/Ic'.format(label[i]))
ax[i].set_xlim([-3,3])
pl.legend()
#pl.tight_layout()
f.close()
(npix,nrand,ncycle,nstokes,nlambda) -> (1, 1, 3, 4, 101)
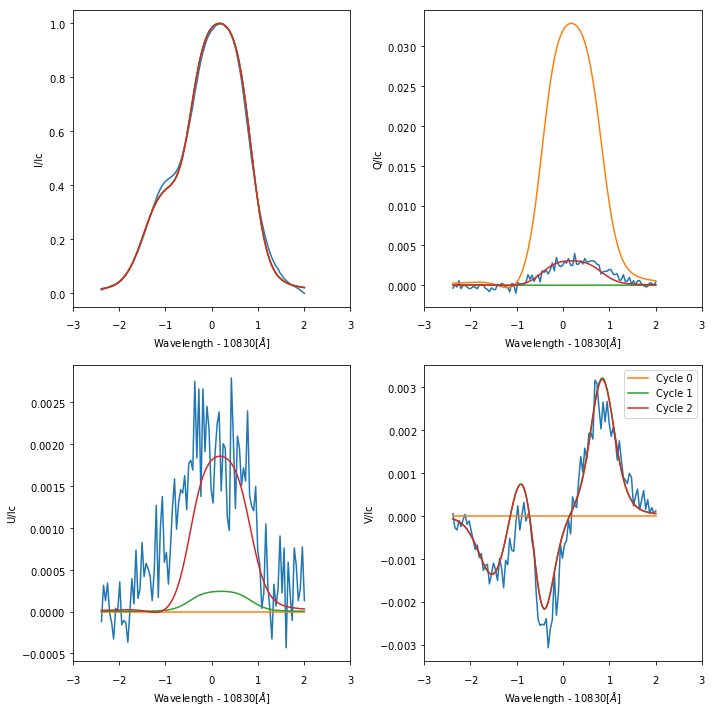
6.3.3. Flare¶
Finally, let us show how one can invert a profile from a flaring region. This profile has been kindly provided by Tetsu Anan and you can find the analysis on http://adsabs.harvard.edu/doi/10.1093/pasj/psy105. There are many things that make Hazel not be physically correct in this scenario, but let’s try to check if we can fit them. Let’s read the profiles and generate the appropriate files.
[2]:
import scipy.io as io
dat = io.readsav('observations/flare.sav')
n_lambda = len(dat['wl'])
noise = np.std(dat['iquv'][1,0:5])
tmp = hazel.tools.File_observation(mode='single')
tmp.set_size(n_lambda=n_lambda, n_pixel=1)
print(tmp.obs['stokes'].shape)
tmp.obs['wavelength'] = dat['wl']
tmp.obs['stokes'][0,:,:] = dat['iquv'].T
tmp.obs['sigma'] = noise*np.ones((n_lambda,4))
tmp.obs['los'][0,:] = np.array([0.0,0.0,90.0])
tmp.obs['boundary'][:] = 0.0
tmp.obs['boundary'][0,:,0] = 1.0
tmp.obs['weights'][:] = 1.0
tmp.save('10830_flare')
(1, 81, 4)
Saving wavelength file : 10830_flare.wavelength
Saving weights file : 10830_flare.weights
Saving 1D Stokes file : 10830_flare.1d
Now we generate an initial file for the atmosphere:
[3]:
tmp = hazel.tools.File_chromosphere(mode='single')
tmp.set_default(n_pixel=1, default='disk')
tmp.save('chromospheres/init_flare')
Setting standard chromosphere
Saving chromospheric 1D model : chromospheres/init_flare.1d
[4]:
%cat conf_flare.ini
# Hazel configuration File
[Working mode]
Output file = output.h5
Number of cycles = 3
# Topology
# Always photosphere and then chromosphere
# Photospheres are only allowed to be added with a filling factor
# Atmospheres share a filling factor if they are in parenthesis
# Atmospheres are one after the other with the -> operator
# Atmosphere 1 = ph2 -> ch1 -> ch2
[Spectral regions]
[[Region 1]]
Name = spec1
#Wavelength = 10826, 10833, 150
Topology = ch1
Stokes weights = 1.0, 1.0, 1.0, 1.0
LOS = 90.0, 0.0, 90.0
Boundary condition = 0.0, 0.0, 0.0, 0.0 # I/Ic(mu=1), Q/Ic(mu=1), U/Ic(mu=1), V/Ic(mu=1)
Wavelength file = '10830_flare.wavelength'
Wavelength weight file = '10830_flare.weights'
Observations file = '10830_flare.1d'
Weights Stokes I = 1.0, 0.1, 0.1, 0.0
Weights Stokes Q = 0.0, 0.0, 1.0, 0.0
Weights Stokes U = 0.0, 0.0, 1.0, 0.0
Weights Stokes V = 0.0, 1.0, 0.1, 0.0
Mask file = None
[Atmospheres]
[[Chromosphere 1]]
Name = ch1 # Name of the atmosphere component
Spectral region = spec1 # Spectral region to be used for synthesis
Height = 3.0 # Height of the slab
Line = 10830 # 10830, 5876
Reference atmospheric model = 'chromospheres/init_flare.1d' # File with model parameters
[[[Ranges]]]
Bx = -2000, 2000
By = -2000, 2000
Bz = -5000, 5000
tau = 0.01, 5.0
v = -10.0, 10.0
deltav = 6.0, 20.0
beta = 0.9, 5.0
a = 0.0, 0.1
ff = 0.0, 1.001
[[[Nodes]]]
Bx = 0, 0, 1
By = 0, 0, 1
Bz = 0, 1, 0
tau = 1, 0, 0
v = 1, 0, 0
deltav = 1, 0, 0
beta = 1, 0, 0
a = 1, 0, 0
ff = 0, 0, 0
And carry out the inversion in two cycles:
[5]:
mod = hazel.Model('conf_flare.ini', working_mode='inversion', verbose=3)
mod.read_observation()
mod.open_output()
mod.invert()
mod.write_output()
mod.close_output()
2018-11-05 09:59:10,915 - Using configuration from file : conf_flare.ini
2018-11-05 09:59:10,918 - Backtracking mode : brent
2018-11-05 09:59:10,919 - Adding spectral region spec1
2018-11-05 09:59:10,920 - - Reading wavelength axis from 10830_flare.wavelength
2018-11-05 09:59:10,922 - - Reading wavelength weights from 10830_flare.weights
2018-11-05 09:59:10,924 - - Using observations from 10830_flare.1d
2018-11-05 09:59:10,925 - - No mask for pixels
2018-11-05 09:59:10,926 - - No instrumental profile
2018-11-05 09:59:10,927 - - Using LOS ['90.0', '0.0', '90.0']
2018-11-05 09:59:10,928 - - Using off-limb normalization (peak intensity)
2018-11-05 09:59:10,928 - - Using boundary condition ['0.0', '0.0', '0.0', '0.0']
2018-11-05 09:59:10,929 - Using 3 cycles
2018-11-05 09:59:10,930 - Not using randomizations
2018-11-05 09:59:10,930 - Adding atmospheres
2018-11-05 09:59:10,931 - - New available chromosphere : ch1
2018-11-05 09:59:10,932 - * Adding line : 10830
2018-11-05 09:59:10,932 - * Magnetic field reference frame : vertical
2018-11-05 09:59:10,934 - * Reading 1D model chromospheres/init_flare.1d as reference
2018-11-05 09:59:10,937 - Adding topologies
2018-11-05 09:59:10,938 - - ch1
2018-11-05 09:59:10,940 - Removing unused atmospheres
2018-11-05 09:59:10,941 - Number of pixels to invert : 1
2018-11-05 09:59:10,943 - Total number of free parameters in all cycles : 8
2018-11-05 09:59:10,979 - -------------
2018-11-05 09:59:10,980 - Cycle 0
2018-11-05 09:59:10,980 - Weights for region spec1 : SI=1.0 - SQ=0.0 - SU=0.0 - SV=0.0
2018-11-05 09:59:10,981 - -------------
2018-11-05 09:59:10,982 - Free parameters for ch1
2018-11-05 09:59:10,983 - - tau with 1 node
2018-11-05 09:59:10,984 - - v with 1 node
2018-11-05 09:59:10,985 - - deltav with 1 node
2018-11-05 09:59:10,985 - - beta with 1 node
2018-11-05 09:59:10,986 - - a with 1 node
/scratch/miniconda3/envs/py36/lib/python3.6/site-packages/scipy/optimize/_minimize.py:763: RuntimeWarning: Method 'bounded' does not support relative tolerance in x; defaulting to absolute tolerance.
"defaulting to absolute tolerance.", RuntimeWarning)
2018-11-05 09:59:12,045 - * Optimal lambda: 0.09613901712208338
2018-11-05 09:59:12,415 - It: 0 - chi2: 55555.56433525851 - lambda: 0.09613901712208338 - rel: -1.9999777778977221
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.430 -0.425 7.307 1.161 0.000
2018-11-05 09:59:13,001 - * Optimal lambda: 0.08247935252073106
2018-11-05 09:59:13,365 - It: 1 - chi2: 20556.398483588408 - lambda: 0.08247935252073106 - rel: -0.9196758185036208
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.203 -1.226 6.511 1.539 0.000
2018-11-05 09:59:14,090 - * Optimal lambda: 0.0661751348188397
2018-11-05 09:59:14,456 - It: 2 - chi2: 10943.3347365054 - lambda: 0.0661751348188397 - rel: -0.6103584230326621
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.083 -2.718 6.192 2.271 0.000
2018-11-05 09:59:14,689 - * Optimal lambda: 0.0015838233632373328
2018-11-05 09:59:14,986 - It: 3 - chi2: 7071.840045349796 - lambda: 0.0015838233632373328 - rel: -0.4298037335785336
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.203 -6.520 6.071 3.266 0.000
2018-11-05 09:59:15,211 - * Optimal lambda: 0.024342561318410814
2018-11-05 09:59:15,500 - It: 4 - chi2: 5298.779563758542 - lambda: 0.024342561318410814 - rel: -0.2866566975005473
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.259 -2.718 6.192 3.474 0.000
2018-11-05 09:59:16,199 - * Optimal lambda: 2.676100841671796
2018-11-05 09:59:16,547 - It: 5 - chi2: 4997.289225073743 - lambda: 2.676100841671796 - rel: -0.05856416557974302
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.259 -2.311 6.511 3.468 0.000
2018-11-05 09:59:17,197 - * Optimal lambda: 1.494491984209709
2018-11-05 09:59:17,571 - It: 6 - chi2: 4344.583429256352 - lambda: 1.494491984209709 - rel: -0.1397376778658725
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.256 -1.661 7.307 3.456 0.000
2018-11-05 09:59:18,326 - * Optimal lambda: 1.1240217523954015
2018-11-05 09:59:18,693 - It: 7 - chi2: 3103.2729058139976 - lambda: 1.1240217523954015 - rel: -0.33333363792136833
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.255 -0.671 9.061 3.445 0.000
2018-11-05 09:59:19,407 - * Optimal lambda: 0.47134769774538293
2018-11-05 09:59:19,765 - It: 8 - chi2: 1687.1669851286654 - lambda: 0.47134769774538293 - rel: -0.5912216635314762
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.257 1.212 12.048 3.441 0.000
2018-11-05 09:59:20,472 - * Optimal lambda: 0.6162592434715304
2018-11-05 09:59:20,757 - It: 9 - chi2: 1360.4395587871902 - lambda: 0.6162592434715304 - rel: -0.21441575323674467
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.272 2.476 13.277 3.480 0.000
2018-11-05 09:59:21,479 - * Optimal lambda: 1.8197967977297405
2018-11-05 09:59:21,757 - It: 10 - chi2: 1349.1810119214601 - lambda: 1.8197967977297405 - rel: -0.008310054173219986
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.274 2.695 13.250 3.488 0.000
2018-11-05 09:59:22,457 - * Optimal lambda: 2.720041425720803
2018-11-05 09:59:22,841 - It: 11 - chi2: 1344.0065367793868 - lambda: 2.720041425720803 - rel: -0.0038426400304497343
Bx By Bz tau v deltav beta a
0.000 0.000 0.000 0.275 2.797 13.184 3.490 0.000
2018-11-05 09:59:23,201 - -------------
2018-11-05 09:59:23,202 - Cycle 1
2018-11-05 09:59:23,202 - Weights for region spec1 : SI=0.1 - SQ=0.0 - SU=0.0 - SV=1.0
2018-11-05 09:59:23,203 - -------------
2018-11-05 09:59:23,204 - Free parameters for ch1
2018-11-05 09:59:23,205 - - Bz with 1 node
2018-11-05 09:59:24,113 - * Optimal lambda: 0.00030736492625848606
2018-11-05 09:59:24,184 - It: 0 - chi2: 260.4803659733832 - lambda: 0.00030736492625848606 - rel: -1.3506201502137916
Bx By Bz tau v deltav beta a
0.000 0.000 1729.585 0.275 2.797 13.184 3.490 0.000
2018-11-05 09:59:24,905 - * Optimal lambda: 0.023925353436515272
2018-11-05 09:59:24,975 - It: 1 - chi2: 252.34132580274837 - lambda: 0.023925353436515272 - rel: -0.031742183691355445
Bx By Bz tau v deltav beta a
0.000 0.000 1966.275 0.275 2.797 13.184 3.490 0.000
2018-11-05 09:59:25,703 - * Optimal lambda: 249.3304218316581
2018-11-05 09:59:25,846 - It: 2 - chi2: 252.34131886461535 - lambda: 249.3304218316581 - rel: -2.749503316444333e-08
Bx By Bz tau v deltav beta a
0.000 0.000 1966.274 0.275 2.797 13.184 3.490 0.000
2018-11-05 09:59:25,987 - -------------
2018-11-05 09:59:25,988 - Cycle 2
2018-11-05 09:59:25,989 - Weights for region spec1 : SI=0.1 - SQ=1.0 - SU=1.0 - SV=0.1
2018-11-05 09:59:25,990 - -------------
2018-11-05 09:59:25,991 - Free parameters for ch1
2018-11-05 09:59:25,992 - - Bx with 1 node
2018-11-05 09:59:25,993 - - By with 1 node
2018-11-05 09:59:26,347 - * Optimal lambda: 9.479695446674016
2018-11-05 09:59:26,484 - It: 0 - chi2: 167.14874325448315 - lambda: 9.479695446674016 - rel: -0.4061720803576269
Bx By Bz tau v deltav beta a
924.234 932.081 1948.851 0.275 2.797 13.184 3.490 0.000
2018-11-05 09:59:27,182 - * Optimal lambda: 0.27653309758863565
2018-11-05 09:59:27,327 - It: 1 - chi2: 159.19817147732678 - lambda: 0.27653309758863565 - rel: -0.048724663345998565
Bx By Bz tau v deltav beta a
136.564 506.619 1948.851 0.275 2.797 13.184 3.490 0.000
2018-11-05 09:59:28,030 - * Optimal lambda: 0.2458829348400783
2018-11-05 09:59:28,264 - It: 2 - chi2: 158.94549989550788 - lambda: 0.2458829348400783 - rel: -0.0015884118060786087
Bx By Bz tau v deltav beta a
48.422 630.237 1948.851 0.275 2.797 13.184 3.490 0.000
2018-11-05 09:59:28,963 - * Optimal lambda: 0.08871830958767633
2018-11-05 09:59:29,190 - It: 3 - chi2: 158.94439473845108 - lambda: 0.08871830958767633 - rel: -6.953080770756462e-06
Bx By Bz tau v deltav beta a
56.350 636.338 1948.851 0.275 2.797 13.184 3.490 0.000
[6]:
f = h5py.File('output.h5', 'r')
print('(npix,nrand,ncycle,nstokes,nlambda) -> {0}'.format(f['spec1']['stokes'].shape))
ncycle = f['spec1']['stokes'].shape[2]
fig, ax = pl.subplots(nrows=2, ncols=2, figsize=(10,10))
ax = ax.flatten()
for i in range(4):
ax[i].plot(f['spec1']['wavelength'][:] - 10830, dat['iquv'][i,:])
for j in range(ncycle):
ax[i].plot(f['spec1']['wavelength'][:] - 10830, f['spec1']['stokes'][0,0,j,i,:], label='Cycle {0}'.format(j))
for i in range(4):
ax[i].set_xlabel('Wavelength - 10830[$\AA$]')
ax[i].set_ylabel('{0}/Ic'.format(label[i]))
ax[i].set_xlim([-3,3])
pl.legend()
#pl.tight_layout()
f.close()
(npix,nrand,ncycle,nstokes,nlambda) -> (1, 1, 3, 4, 81)
/scratch/miniconda3/envs/py36/lib/python3.6/site-packages/matplotlib/figure.py:2362: UserWarning: This figure includes Axes that are not compatible with tight_layout, so results might be incorrect.
warnings.warn("This figure includes Axes that are not compatible "
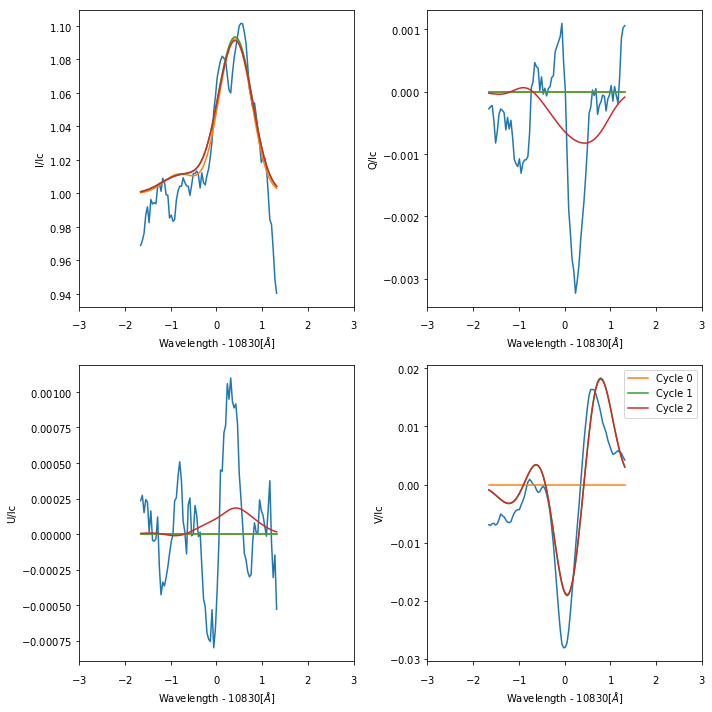
[ ]: